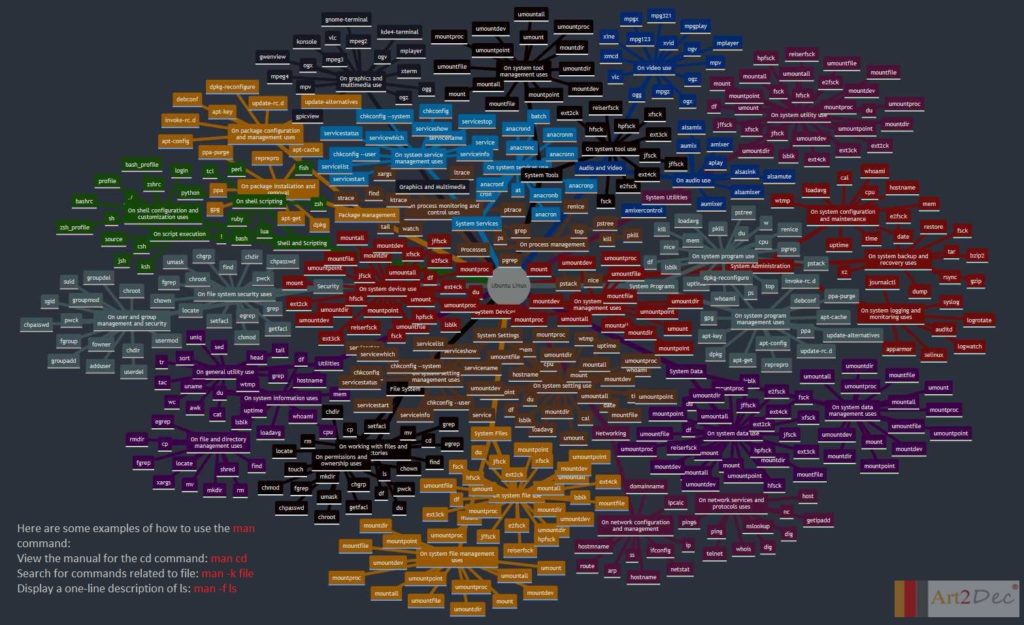
Main macOS Sonoma 14.5 commands.
Main Windows PowerShell Commands.
Main Windows cmd prompt commands.
Docker Best practices.
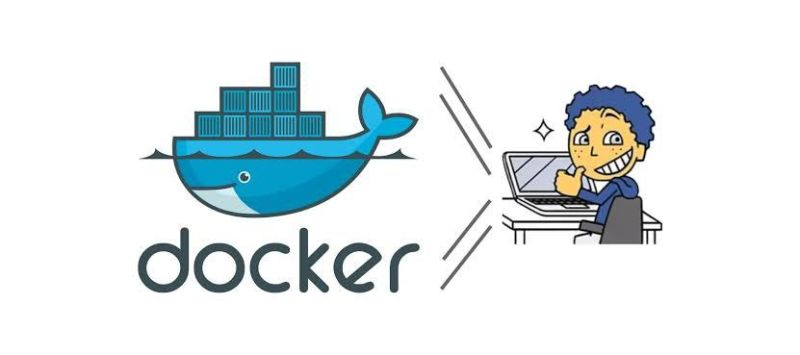
Docker has revolutionized the world of containerization, enabling scalable and efficient application deployment.
To make the most of this powerful tool, here are 10 essential Docker best practices:
𝗦𝘁𝗮𝗿𝘁 𝘄𝗶𝘁𝗵 𝗮 𝗟𝗶𝗴𝗵𝘁𝘄𝗲𝗶𝗴𝗵𝘁 𝗕𝗮𝘀𝗲 𝗜𝗺𝗮𝗴𝗲: Use minimalist base images to reduce container size and vulnerabilities.
𝗦𝗶𝗻𝗴𝗹𝗲 𝗣𝗿𝗼𝗰𝗲𝘀𝘀 𝗽𝗲𝗿 𝗖𝗼𝗻𝘁𝗮𝗶𝗻𝗲𝗿: Keep it simple – one process per container for better isolation and maintainability.
𝗨𝘀𝗲 𝗗𝗼𝗰𝗸𝗲𝗿 𝗖𝗼𝗺𝗽𝗼𝘀𝗲: Define multi-container applications in a YAML file for easy management.
𝗩𝗼𝗹𝘂𝗺𝗲 𝗠𝗼𝘂𝗻𝘁𝗶𝗻𝗴: Store data outside the container to preserve it, even if the container is removed.
𝗖𝗼𝗻𝘁𝗮𝗶𝗻𝗲𝗿 𝗢𝗿𝗰𝗵𝗲𝘀𝘁𝗿𝗮𝘁𝗶𝗼𝗻: Consider Kubernetes or Docker Swarm for managing containers at scale.
𝗩𝗲𝗿𝘀𝗶𝗼𝗻𝗶𝗻𝗴 𝗮𝗻𝗱 𝗧𝗮𝗴𝗴𝗶𝗻𝗴: Always tag images with version numbers to ensure reproducibility.
𝗛𝗲𝗮𝗹𝘁𝗵 𝗖𝗵𝗲𝗰𝗸𝘀: Implement health checks to monitor container status and reliability.
𝗥𝗲𝘀𝗼𝘂𝗿𝗰𝗲 𝗟𝗶𝗺𝗶𝘁𝘀: Set resource constraints to prevent one container from hogging resources.
𝗗𝗼𝗰𝗸𝗲𝗿𝗳𝗶𝗹𝗲 𝗕𝗲𝘀𝘁 𝗣𝗿𝗮𝗰𝘁𝗶𝗰𝗲𝘀: Optimize Dockerfiles by minimizing layers and using caching effectively.
𝗦𝗲𝗰𝘂𝗿𝗶𝘁𝘆: Regularly update images, scan for vulnerabilities, and follow security best practices.
Install Docker on Linux with a single command.
Run this to install Docker:
$ curl -fsSL get.docker.com | sh
To preview the installation steps first:
$ curl -fsSL get.docker.com -o get-docker.sh
$ sudo sh ./get-docker.sh –dry-run
How to Send Email using Shell Script (gmail).
In this article, we will learn how to send email using shell scripting. Sending emails programmatically can be very useful for automated notifications, error alerts, or any other form of communication that needs to be sent without manual intervention. Shell scripting offers a powerful way to achieve this through various utilities and tools available in Unix-like operating systems.
Prerequisites
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- Basic knowledge of Shell scripting.
Step #1:Generate the App Password
First go to your account:
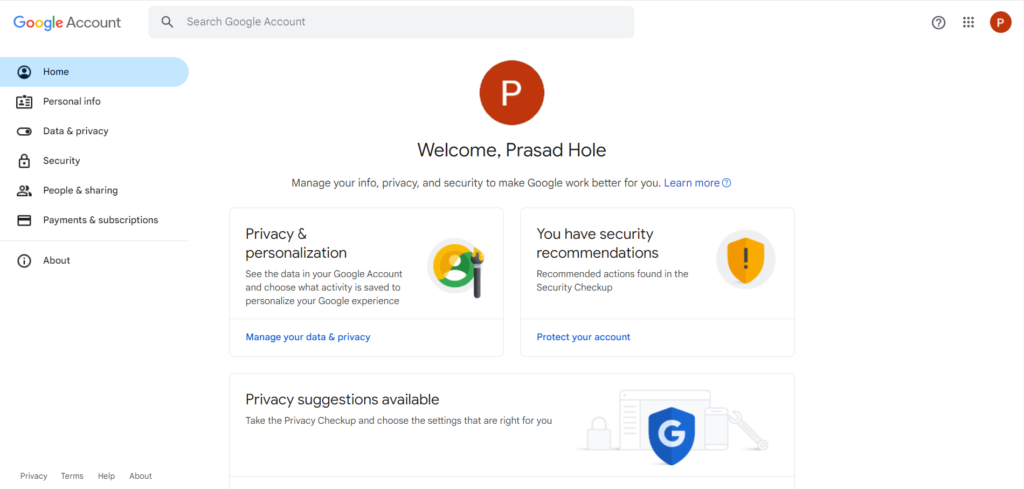
Search for App Password in the search bar.
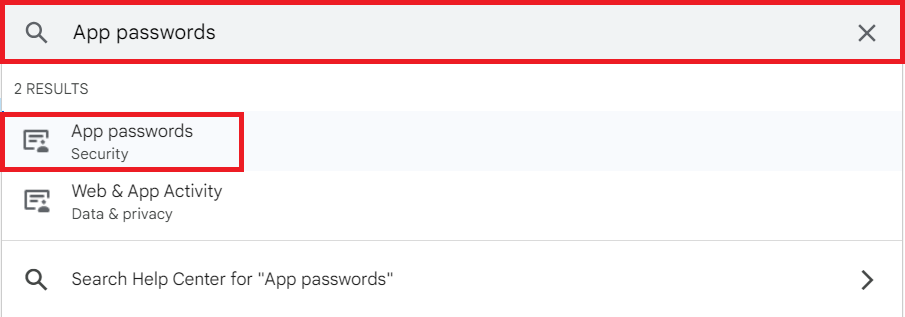
Enter your google account password to verify it’s you:
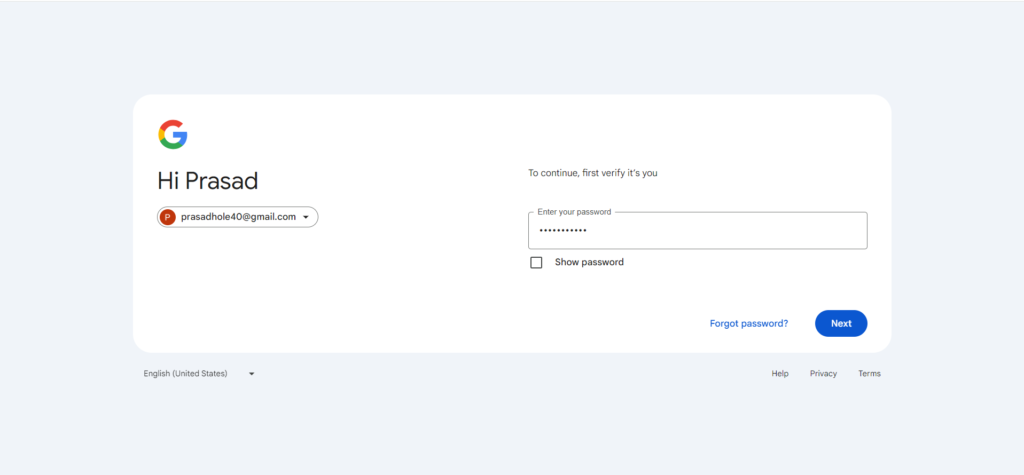
Now Enter the app name for which you wanted to get the app password like Shell Script.
And click on Create to create it:
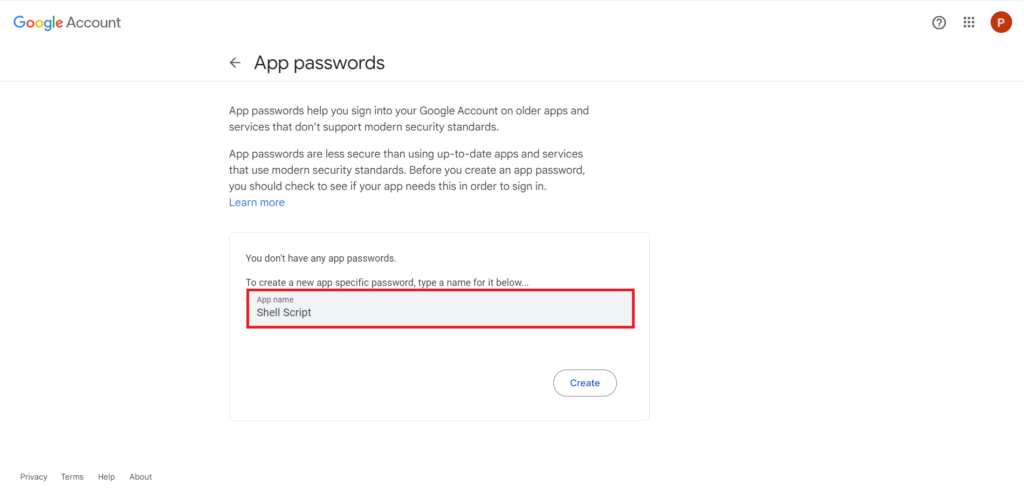
the password will be generated. Note it down cause will be using it.
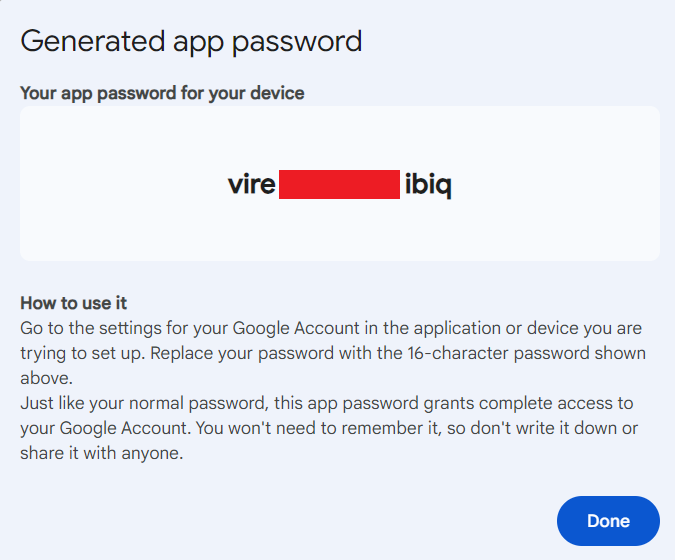
Step #2:Create a file in Ubuntu
Open the terminal and use the nano command to create a new file:
nano email.sh
Step #3:Write a Script to Send Emails
Below is a basic script to send an email.
-----------------------------------------
#!/bin/bash
# Prompt the user for input
read -p "Enter your email: " sender
read -p "Enter recipient email: " receiver
read -s -p "Enter your Google App password: " gapp
echo
read -p "Enter the subject of mail: " sub
# Read the body of the email
echo "Enter the body of mail (Ctrl+D to end):"
body=$(</dev/stdin)
# Sending email using curl
response=$(curl -s --url 'smtps://smtp.gmail.com:465' --ssl-reqd \
--mail-from "$sender" \
--mail-rcpt "$receiver" \
--user "$sender:$gapp" \
-T <(echo -e "From: $sender\nTo: $receiver\nSubject: $sub\n\n$body"))
if [ $? -eq 0 ]; then
echo "Email sent successfully."
else
echo "Failed to send email."
echo "Response: $response"
fi
------------------------------------------
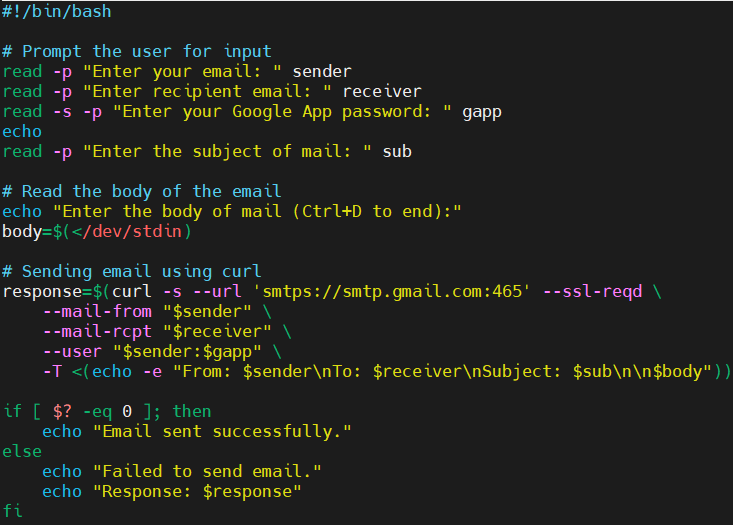
Save the file and exit the editor.
Explanation of the Script:
This script is designed to send an email using Gmail’s SMTP server through the command line. Here’s a step-by-step explanation of each part of the script:
- Shebang: The
#!/bin/bash
line at the beginning of the script indicates that the script should be run using the Bash shell. - User Input Prompts: These lines prompt the user to enter
- The sender’s email address.
- The recipient’s email address.
- The sender’s Google App password (entered silently using
-s
to hide the input). - The subject of the email.
- Reading the Email Body: This section prompts the user to enter the body of the email. The user can type the email content and end input by pressing
Ctrl+D
. The body of the email is then captured into the variablebody
. - Sending the Email: This part of the script uses
curl
to send the email-s
makescurl
run silently.--url 'smtps://smtp.gmail.com:465'
specifies the Gmail SMTP server with SSL on port 465.--ssl-reqd
ensures SSL is required.--mail-from "$sender"
specifies the sender’s email address.--mail-rcpt "$receiver"
specifies the recipient’s email address.--user "$sender:$gapp"
provides the sender’s email and Google App password for authentication.-T <(echo -e "From: $sender\nTo: $receiver\nSubject: $sub\n\n$body")
sends the email content including headers and body.
- Handling the Response: This final part checks the exit status of the
curl
command ($?
):- If the exit status is
0
, it prints “Email sent successfully.” - If the exit status is not
0
, it prints “Failed to send email.” and outputs the response fromcurl
.
- If the exit status is
Step #4:Make file executable
Change the file permissions to make it executable using the chmod command:
chmod +x email.sh
Step #5:Run the script
Run the script by executing the following command:
./email.sh
You will be prompted to enter the sender’s email, recipient’s email, Google App password, subject, and body of the email:
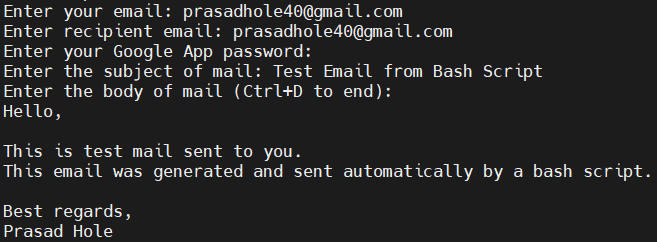
You will get the message Email sent successfully.
Now check the mail box to see if you receive the mail or not.
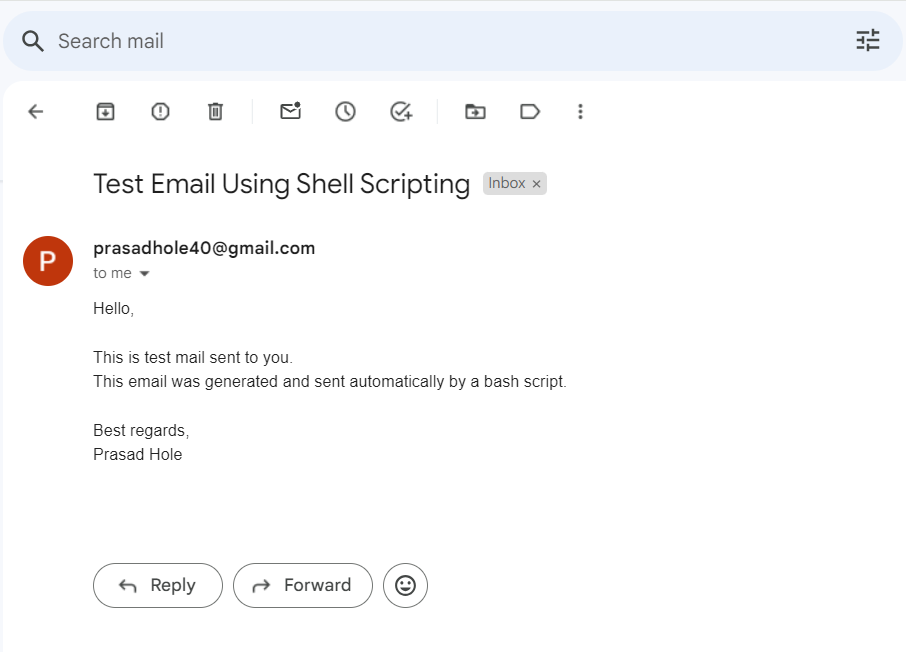
Conclusion:
In conclusion, sending emails using shell scripting can greatly enhance your automation tasks, enabling seamless communication and notifications. This approach is especially useful for automated notifications, error alerts, and other routine communications. With the power of shell scripting, you can integrate emails functionality into your workflows, ensuring timely and efficient information exchange.
Shell Script to send email if multiple websites are down (gmail).
In this article, we will learn Shell Script to send email if website down, how to automate the process of checking the status of URLs and sending email notifications if any of them are down. When a website goes down or experiences issues, it’s essential to be promptly notified so that necessary actions can be taken to restore its functionality.
One effective way to achieve this is by automating email notifications to alert system administrators or stakeholders about the status of URLs. We’ll utilize shell scripting to create a simple yet effective solution for monitoring website health and ensuring timely notifications in case of any disruptions.
Prerequisites
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- Basic knowledge of Shell scripting.
Step #1:Generate the App Password
First go to your account.
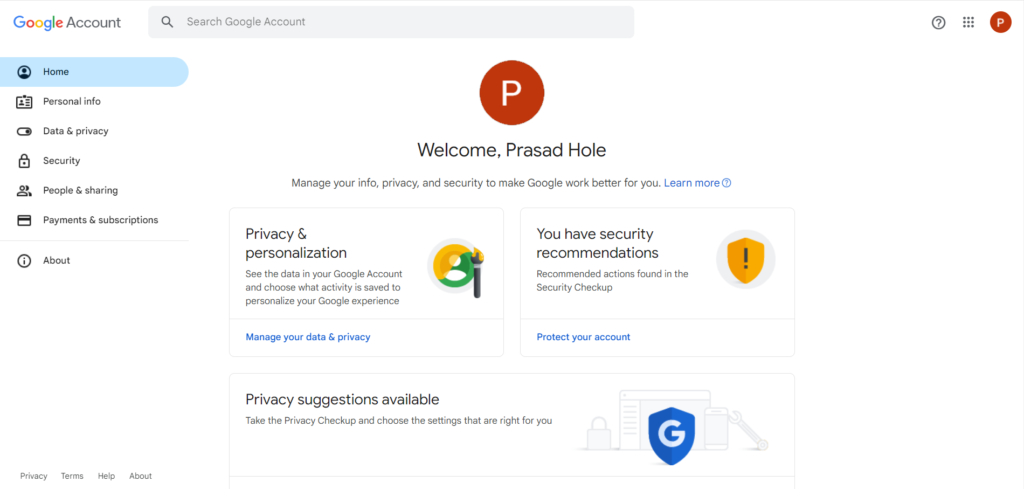
Search for App Password in the search bar.
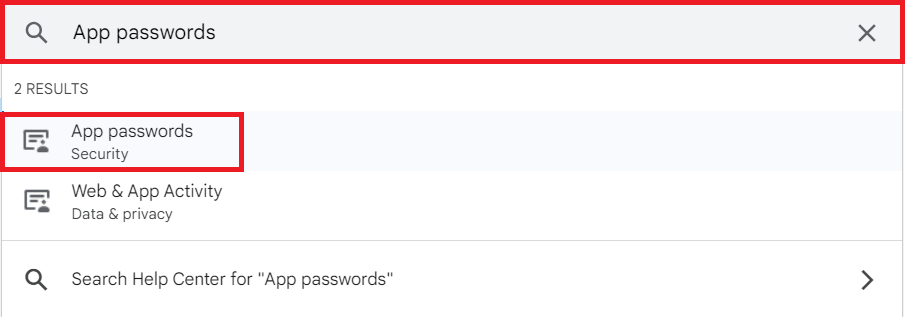
Enter your google account password to verify it’s you.
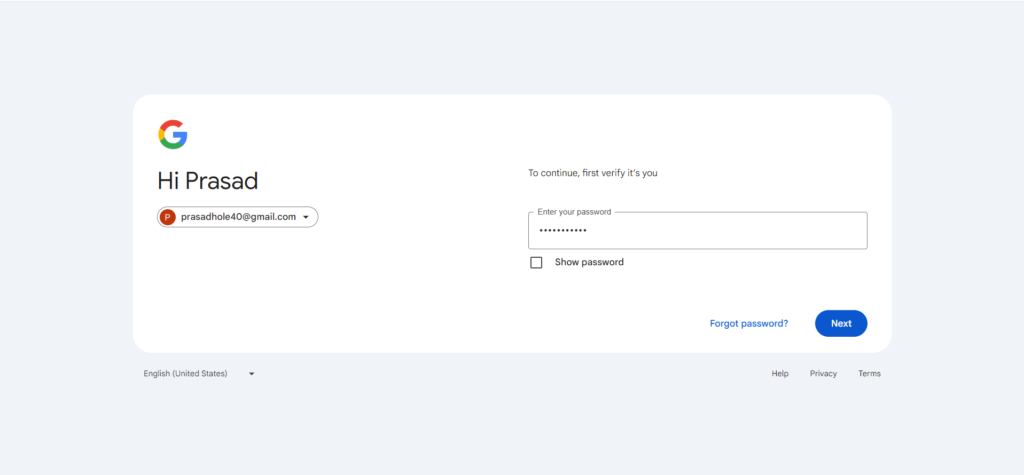
Now Enter the app name for which you wanted to get the app password like URL Notification.
And click on Create to create it
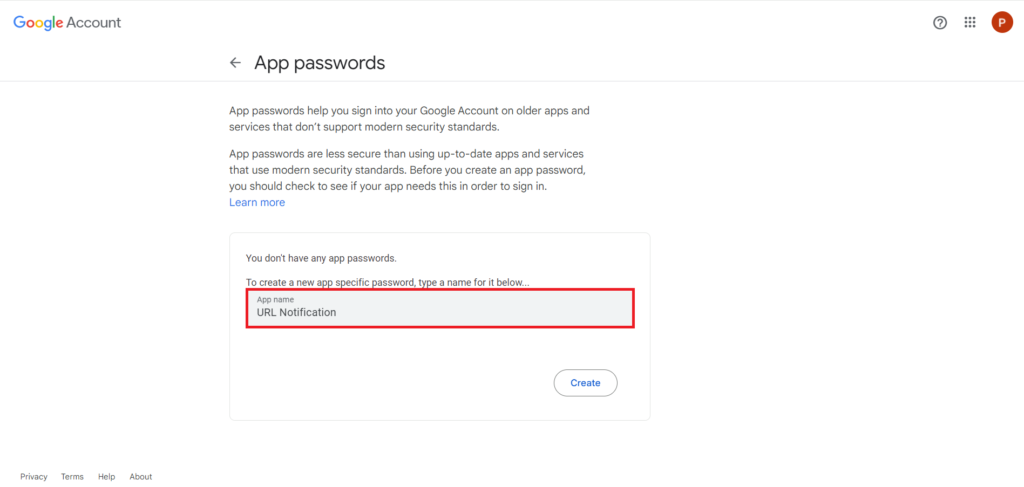
the password will be generated. Note it down cause will be using it
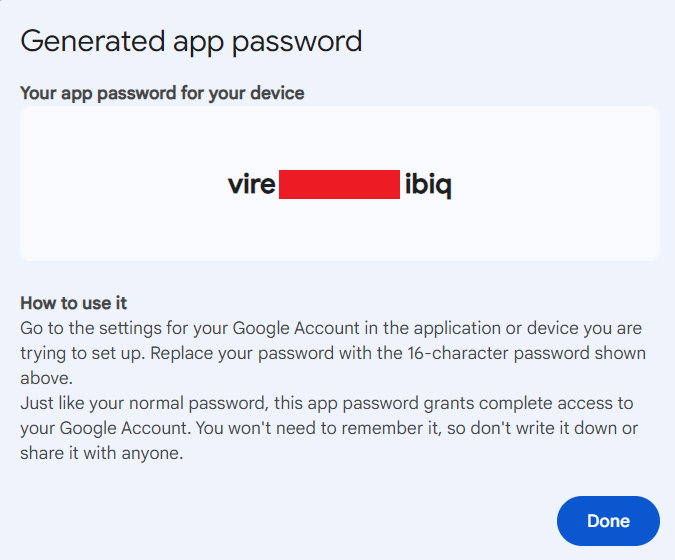
Step #2:Create a file in Ubuntu
Open the terminal and use the nano command to create a new file.
nano url_notify.sh
----------------------------------------------------
Step #3:Write a Script to Send Email Notification
Below is a basic script to send an email notification if any of the url’s are down:
----------------------------------------------------
#!/bin/bash
# Prompt the user for input
read -p "Enter your email: " sender
read -p "Enter recipient email: " receiver
read -s -p "Enter your Google App password: " gapp
echo
# List of URLs to check
urls=("https://www.example.com" "https://www.google.com" "https://www.openai.com")
# Function to check the status of URLs and send email notification if any are down
check_urls_and_send_email() {
local down_urls=""
local subject="Website Down"
for url in "${urls[@]}"; do
response=$(curl -Is "$url" | head -n 1)
if [[ ! $response =~ "200" ]]; then
down_urls+="$url\n"
fi
done
if [[ -n $down_urls ]]; then
body="The following websites are down:\n\n$down_urls"
email_content="From: $sender\nTo: $receiver\nSubject: $subject\n\n$body"
response=$(curl -s --url 'smtps://smtp.gmail.com:465' --ssl-reqd \
--mail-from "$sender" \
--mail-rcpt "$receiver" \
--user "$sender:$gapp" \
-T <(echo -e "$email_content"))
if [ $? -eq 0 ]; then
echo "Email sent successfully."
else
echo "Failed to send email."
echo "Response: $response"
fi
else
echo "All websites are up."
fi
}
# Call the function to check URLs and send email
check_urls_and_send_email
-------------------------------------------------
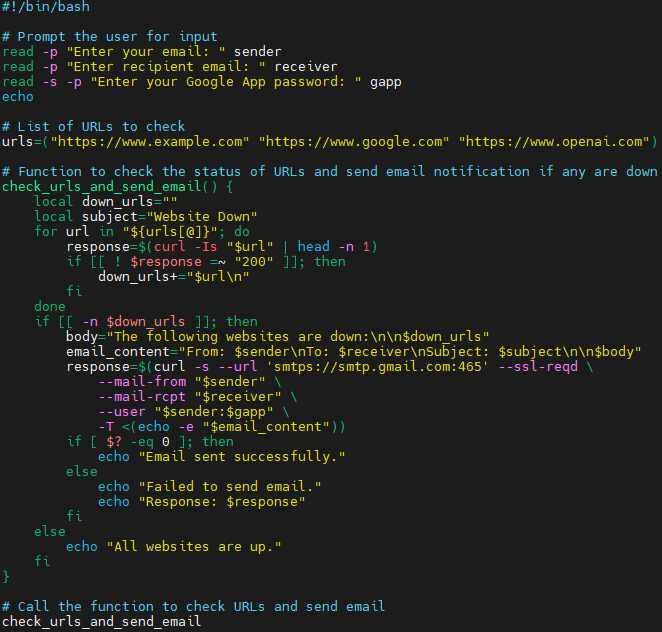
Save the file and exit the editor.
Explanation of the Script:
- User Input Prompt:
- The script prompts the user to enter their email address (sender), recipient’s email address (receiver), and Google App password (gapp).
- List of URLs:
- The script defines an array urls containing the URLs to be checked for their status. These URLs represent the websites whose availability we want to monitor.
- Function to Check URLs and Send Email:
- The check_urls_and_send_email() function iterates through each URL, using curl to check their status. If any URLs are found to be down, it constructs and sends an email notification containing details of the down URLs to the specified recipient. The email is sent using SMTPS protocol with SSL, and the script handles the response to determine the success of the email sending operation.
- Function Call:
- The script calls the check_urls_and_send_email function to initiate the process of checking URL statuses and sending email notifications.
Step #4:Make file executable
Change the file permissions to make it executable using the chmod command.
chmod +x url_notify.sh
Step #5:Run the script
Run the script by executing the following command.
./url_notify.sh
You will be prompted to enter the sender’s email, recipient’s email and Google App password:

You will receive the message Email sent successfully.
Now check the mail box to verify if you received the mail or not.
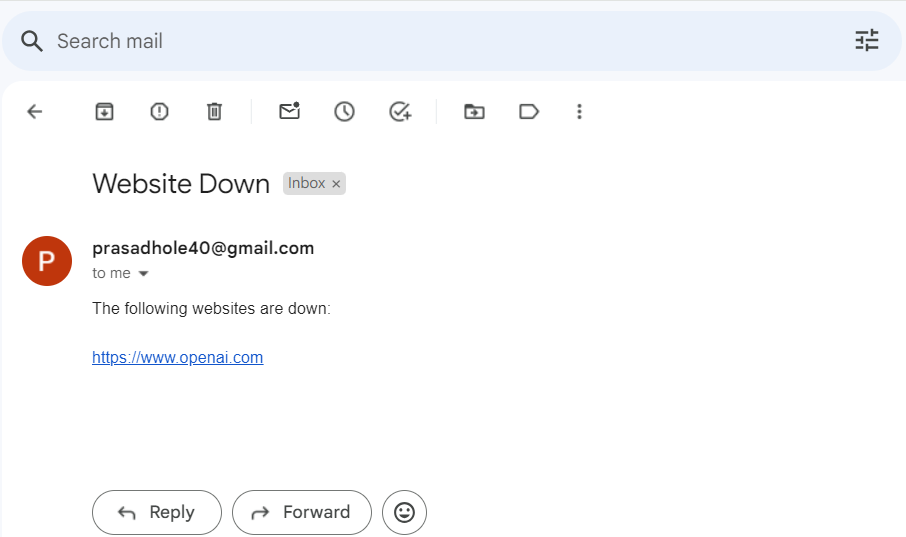
Conclusion:
In conclusion, automating email notifications for URL status monitoring using shell scripting is a proactive approach to ensuring the continuous availability and performance of critical web services and applications. By implementing a simple shell script, organizations can establish an efficient mechanism for monitoring URL health and receiving timely alerts in case of any disruptions. By following the steps outlined in this article, organizations can enhance their website monitoring capabilities and minimize downtime, ultimately improving the overall reliability and user experience of their online services.
AWS Services cheatsheet.

Get Started with Ubuntu 24.04: Beginner-Friendly Installation & Tweaks.
Ubuntu 24.04 LTS, codename Noble Numbat, was released on April 25, 2024, and is now available for download from the official Ubuntu website and its mirrors. This release will receive official security and maintenance updates for five years, until April 2029.
Some key points about Ubuntu 24.04 LTS:
- Release date: April 25, 2024
- Codename: Noble Numbat
- Support period: 5 years (until April 2029)
- Download from: https://ubuntu.com/download/desktop or official mirrors
Ubuntu has long been recognized as one of the most popular and widely used Linux distributions among desktop users.
However, it has experienced fluctuations in its popularity, particularly during the period when it introduced significant changes to its desktop experience with the user interface.
A key feature of this new Ubuntu release is that it comes out at the same time (or almost the same time) as other popular versions like Kubuntu, Lubuntu, Xubuntu, and Ubuntu Studio.
This gives users more options for desktop environments, all with official support. The support period can differ (usually 3 years for non-LTS and 5 years for LTS versions), but releasing them together makes it easier for both individuals and companies to choose.
Ubuntu 24.04 Features
Here are some noticeable changes in Ubuntu 24.04 (Noble Numbat):
- Modern Desktop – Ubuntu 24.04 utilizes GNOME 46, offering a refined interface, improved multitasking, and better performance.
- Revamped Installer – The installer boasts a fresh look, improved accessibility, and even supports easier deployment for businesses.
- Upgraded App Center – The App Center features a new Flutter-based interface for smoother navigation, clearer categories, and user ratings.
- Enhanced Security – As an LTS release, Ubuntu 24.04 prioritizes stability with the latest Linux kernel 6.8 for improved security and hardware compatibility.
- Window Tiling Assistant – GNOME 46 introduces advanced window tiling, allowing efficient snapping of windows to various screen sections, including corners.
- Granular Update Control – Separate stability and cutting-edge updates offer users more control over what gets installed.
- Year 2038 Fix (ARM) – The year 2038 issue is addressed on ARM architecture, ensuring accurate timekeeping for future users.
- Minimal Default Installation – The default Ubuntu Desktop installation is now minimal, with options to add applications like LibreOffice or Thunderbird.
Ubuntu has one of the most simple and straightforward installers among all Linux distributions which makes the job of installing the system on hardware very easy even for a beginner or an uninitiated Linux or Windows user with just a few clicks.
System Requirements
Following are the minimum system requirements for installing Ubuntu 24.04 Desktop:
- A 2 GHz dual-core processor
- 4 GB of RAM
- 25 GB of free hard drive space.
This tutorial will cover fresh installation of Ubuntu 24.04 and with a basic walk through and a few system tweaks and applications.
Step 1: Installing Ubuntu 24.04 Desktop
1. Download the Ubuntu ISO image from the Ubuntu website, and then use a USB Linux Installer to burn the ISO to a USB stick.
2. After creating a bootable Ubuntu USB drive, restart your computer and go into the BIOS or boot menu by pressing the designated key (usually F2
, F12
, or Del
) during startup. Choose the USB drive as the boot device and press Enter.
3. The USB content is loaded into your RAM memory until it reaches the installation screen where you will be given the option to “Try Ubuntu” or “Install Ubuntu“.
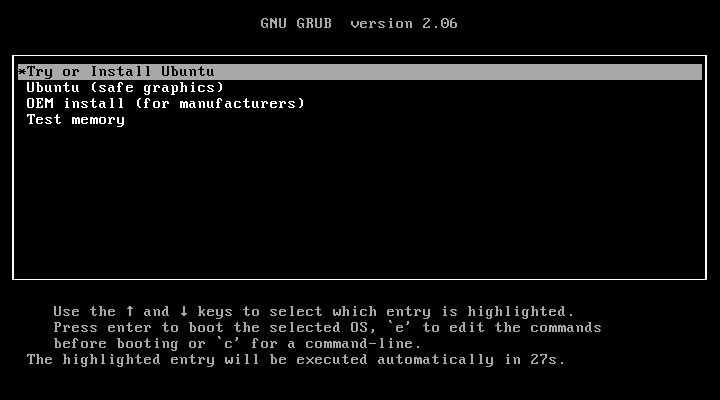
4. The next step asks you to test Ubuntu before installing it, choose “Try Ubuntu“, which allows you to use Ubuntu from the USB drive without making any changes to your computer’s hard drive.
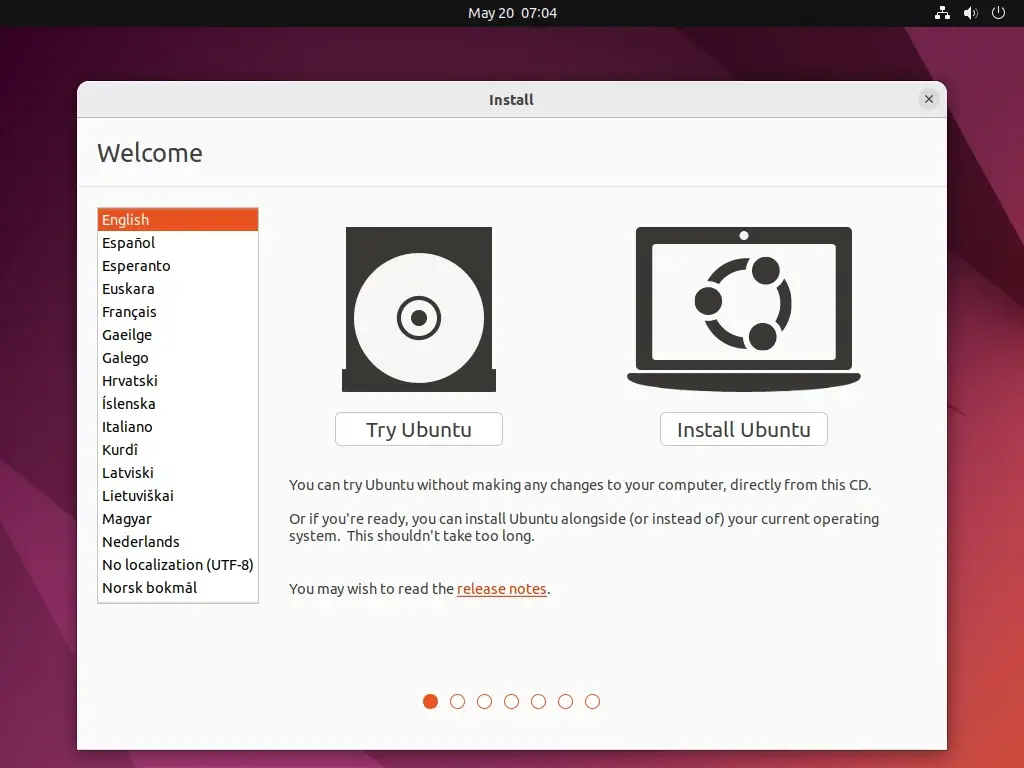
5. If you’re ready to install Ubuntu, select “Install Ubuntu” and follow the on-screen instructions to select your language, and keyboard layout.
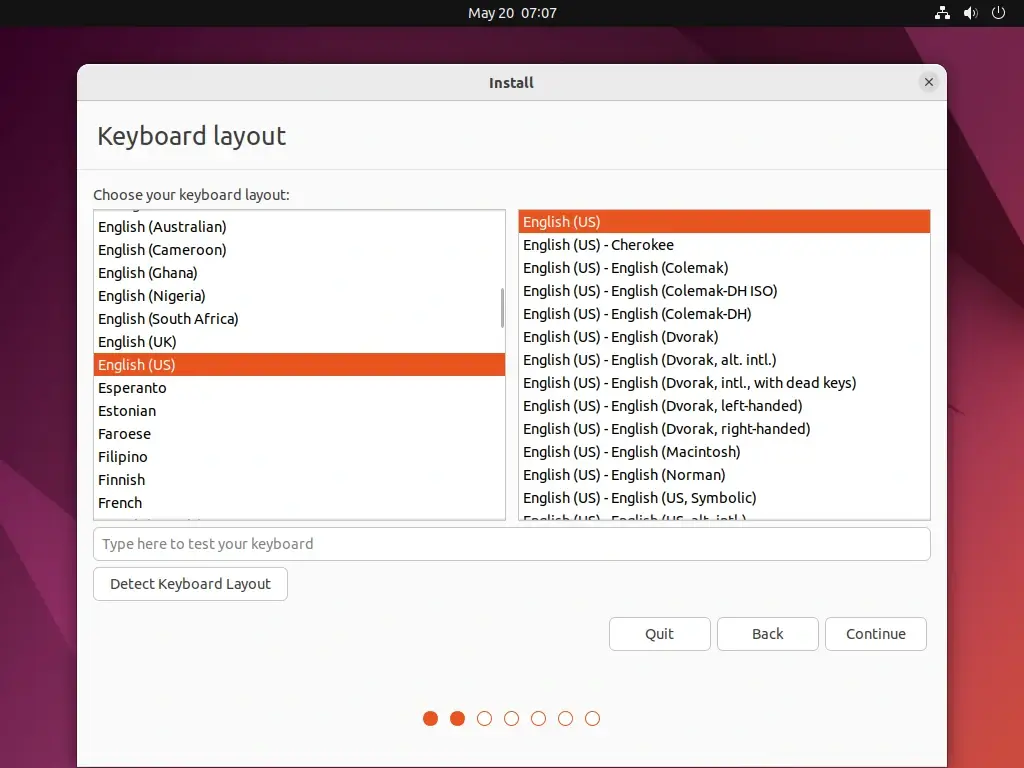
6. Next, choose “Normal installation” to install the full Ubuntu desktop with office software, games, and media players. You will also have the option to download updates and third-party software during the installation process.
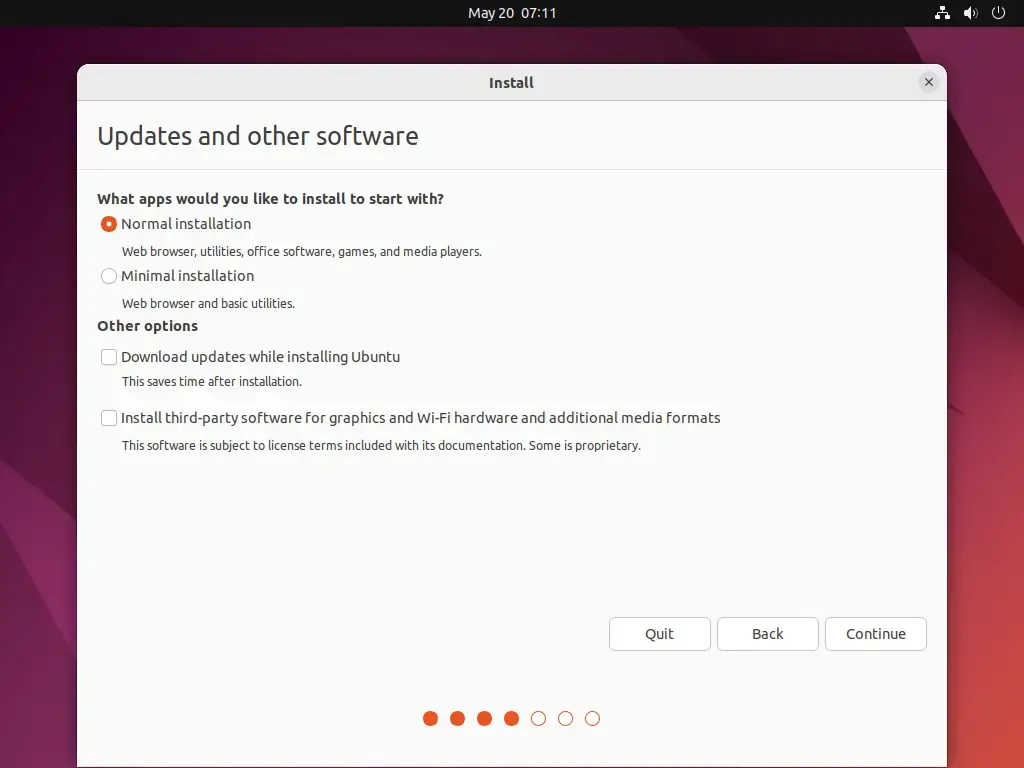
7. Ubuntu will prompt you to choose how you want to partition your disk. You can select “Erase disk and install Ubuntu” to use the entire disk or choose “Something else” for manual partitioning.
If you’re new to partitioning, it’s recommended to select the “Erase disk and install Ubuntu” option.
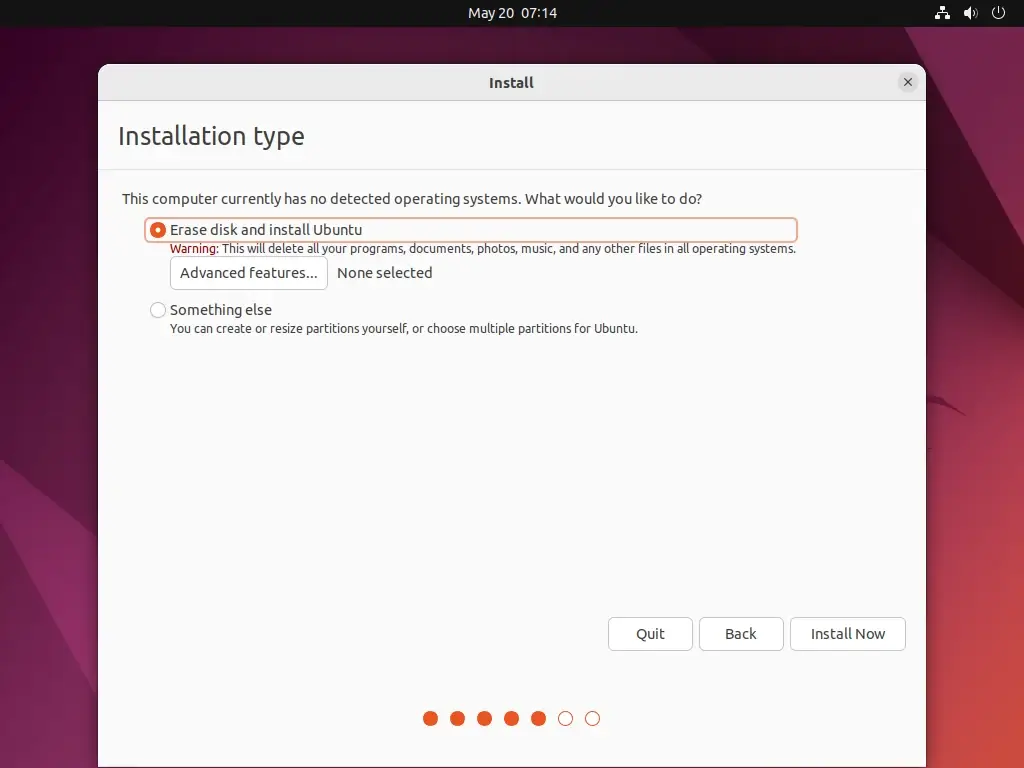
8. After your disk has been sliced hit on Install Now button. In the next stage choose your Location from the map – Location will have an impact on your system time also so be advised to choose your true location.
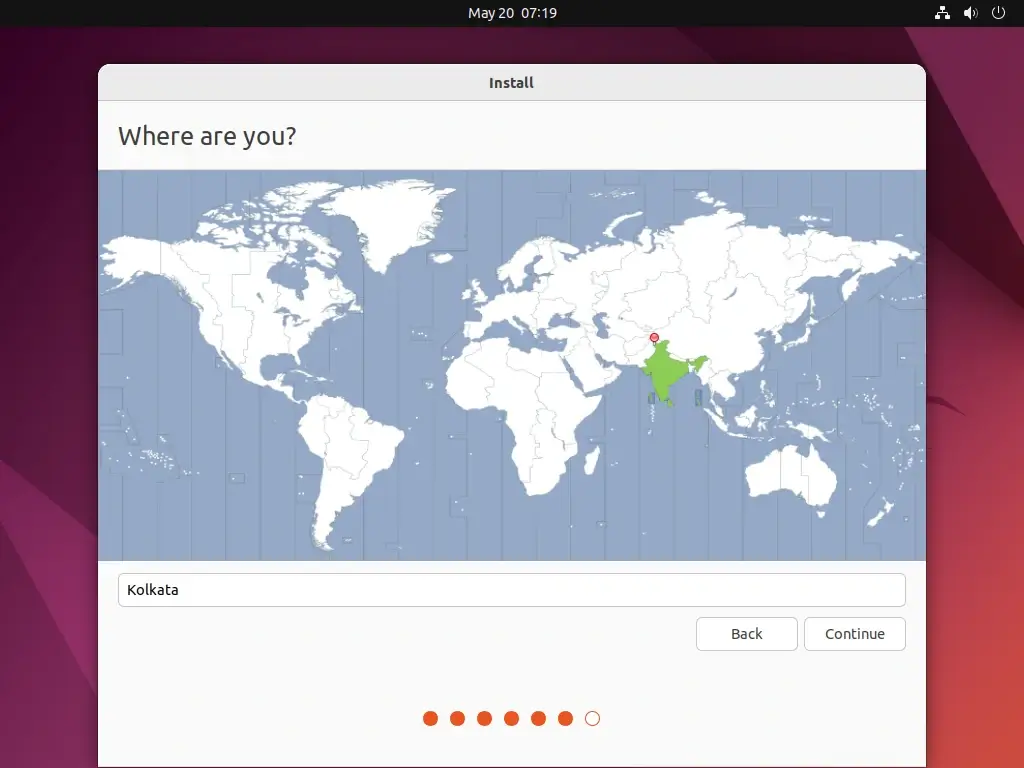
9. Next, enter your name, desired username, password, and computer name to create a user account for Ubuntu.
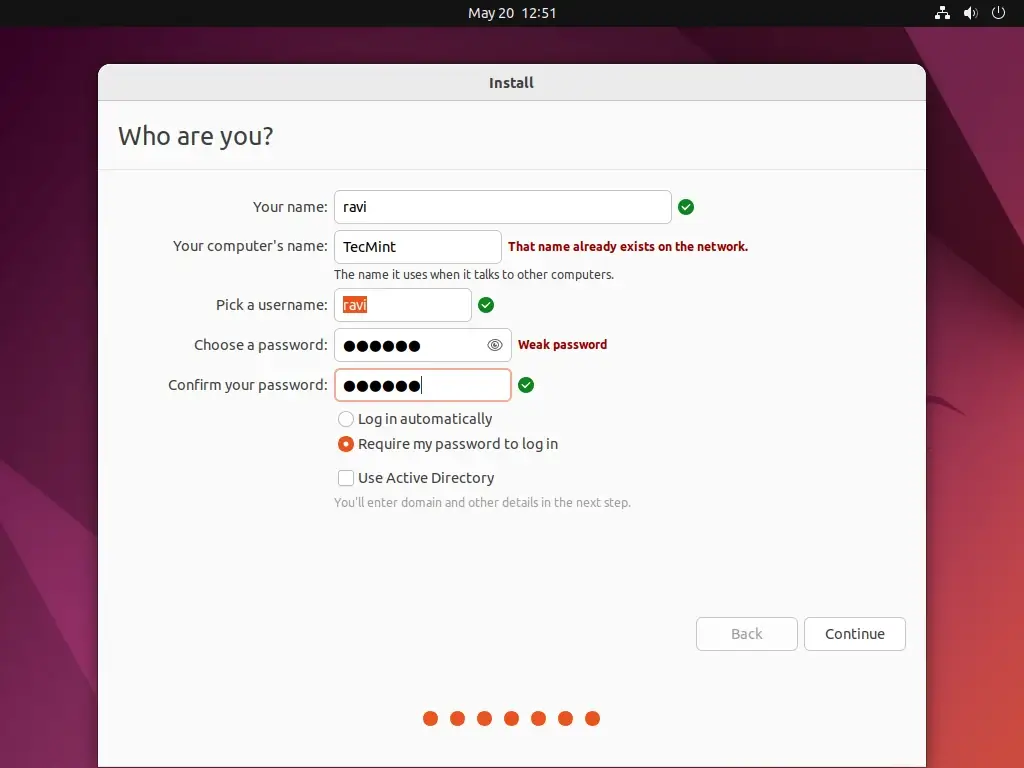
10. Review your settings and click “Continue” to begin the installation process.
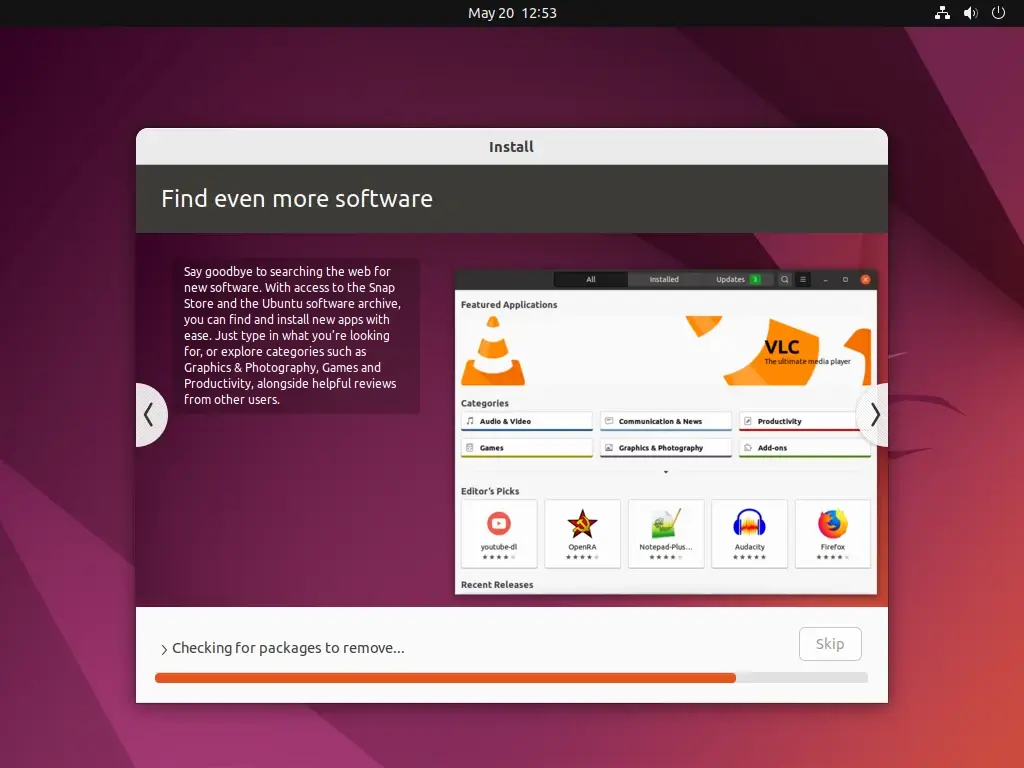
The installer now starts copying system files to your hard drive while it presents you with some information about your brand new Ubuntu LTS for 5 years support system.
After the installer finishes his job click on Restart Now prompt and press Enter after a few seconds for your system to reboot.
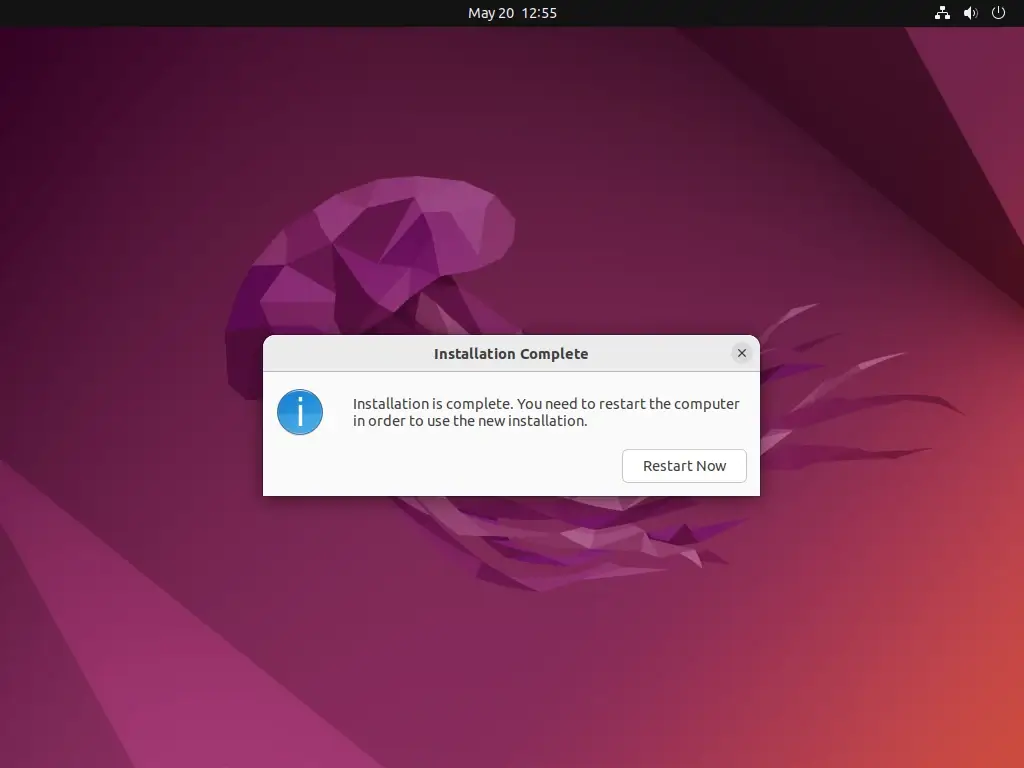
Congratulations!! Ubuntu 24.04 is now installed on your machine and is ready for daily usage.
Step 2: System Update and Basic Software
After first logging into your new system is time to review your software sources to ensure you have all necessary repositories enabled, including main, universe, restricted, and multiverse.
Check for Updates
Open “Software & Updates” from the applications menu, go to the “Ubuntu Software” tab, and ensure that all the checkboxes for the Ubuntu repositories are checked.
You may also check the “Source Code” option if you want access to source packages.
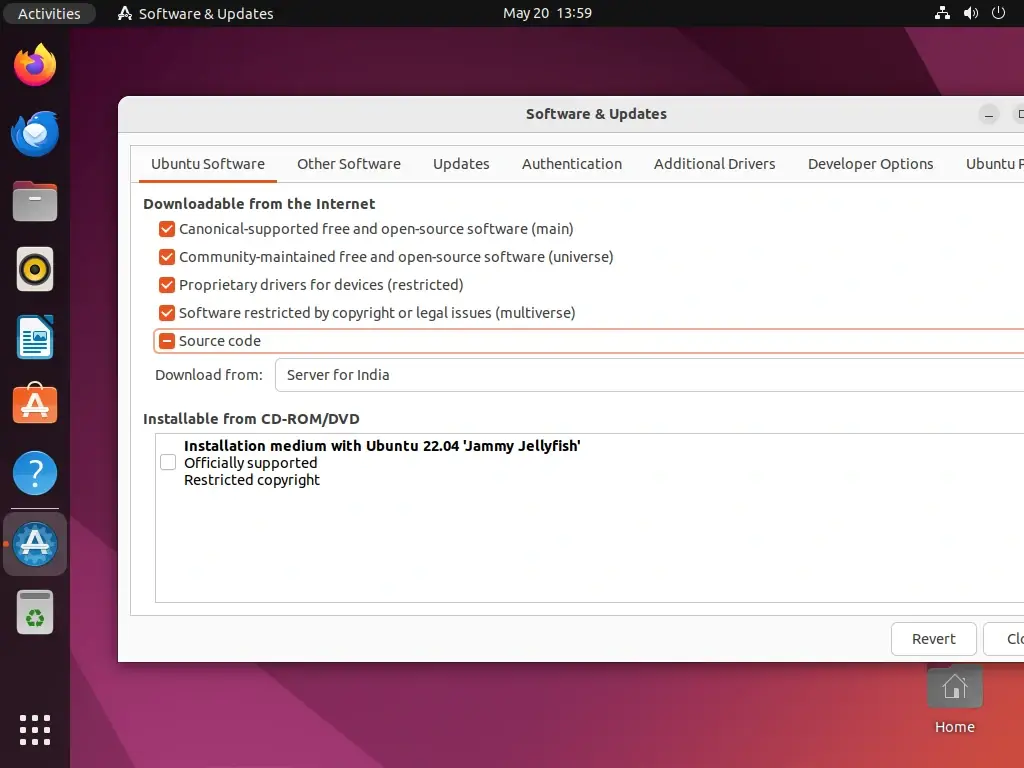
After making any changes to the software sources, click “Close” and then “Reload” to update the software repositories.
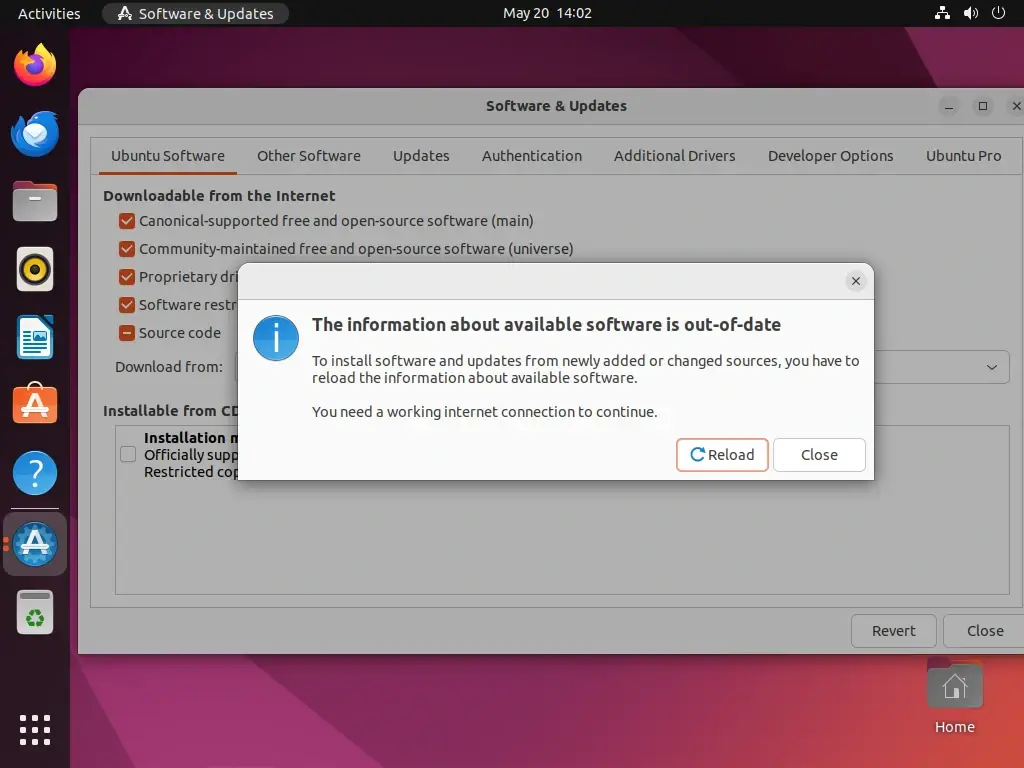
Next, open a terminal and issue the following commands to keep your system secure and protected against potential vulnerabilities.
sudo apt-get update
sudo apt-get upgrade
Install Basic Software
For basic user usage, type “Ubuntu Software” in the search bar, press Enter to open it, and browse through the search results to find the software you’re looking for.
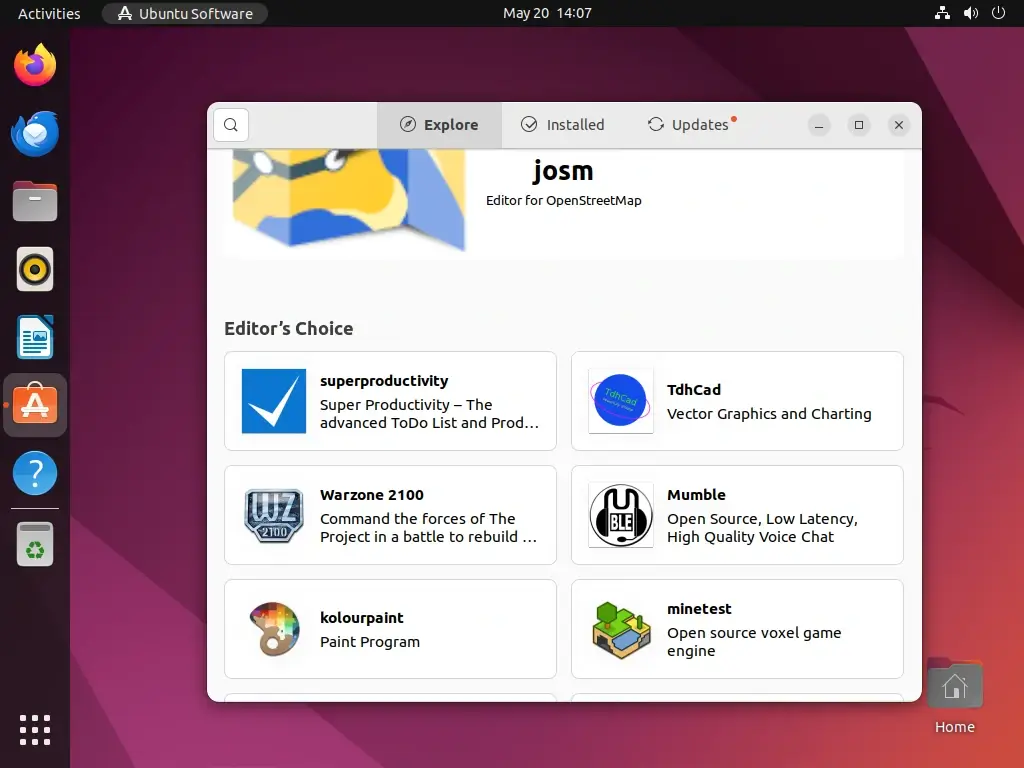
Enable ‘Minimize on Click
If you prefer to minimize windows by clicking on the app icon, you can enable this feature using a simple command in the terminal:
gsettings set org.gnome.shell.extensions.dash-to-dock click-action 'minimize'
Install GNOME Tweaks
GNOME Tweaks is a powerful tool that allows for extensive customization options such as changing themes, fonts, window behavior, and more.
You can install GNOME Tweaks through the Ubuntu Software app and access it from the applications grid by searching for “tweaks“.
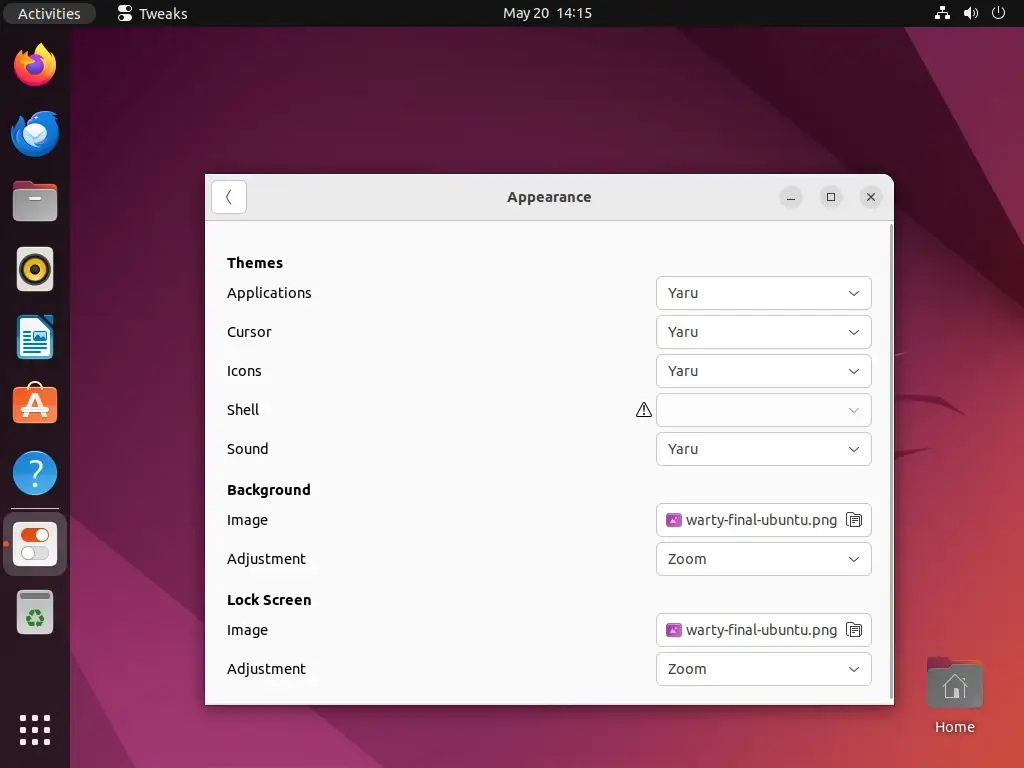
Enable Night Light
You might want to turn on the “night light” setting in GNOME, which helps to lower the amount of blue light that comes from your screen. This can be good for your sleep. You can find this setting in the “Screen Display” part of your Settings.
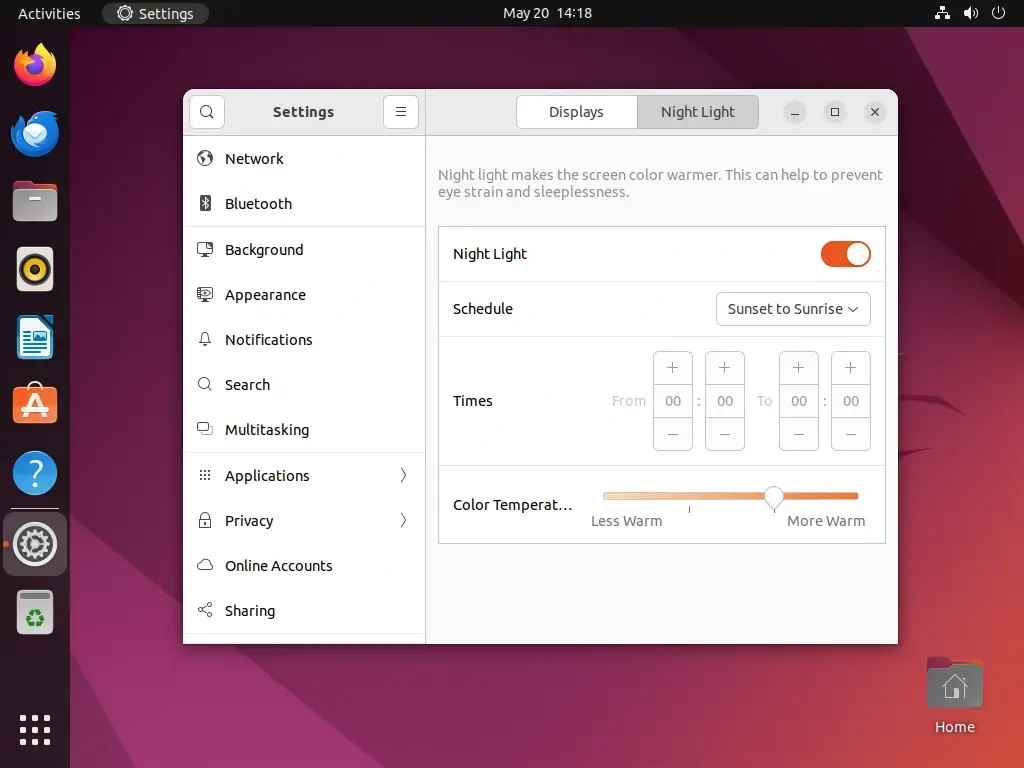
Customize Desktop Environment
Ubuntu offers different desktop environments like GNOME, KDE, and Xfce. You can install and switch between these environments based on your preferences using terminal commands as shown.
Install KDE on Ubuntu:
sudo apt install kubuntu-desktop
Install Xfce on Ubuntu:
sudo apt install xubuntu-desktop
Install Snap Packages
You might want to try installing Snap packages, a new way of packaging software for Linux. It lets you get lots of different software applications and tools easily.
sudo apt install snapd
That’s all for basic Ubuntu installation and minimal software required for average users to browse the Internet, instant messaging, listen to music, watch movies, YouTube clips, or write documents.